Sometimes your application wants to send e-mail notifications to customers. You need to let the customers of your application to set the e-mail server that the application uses to send such e-mail notifications. As discussed in the previous article How to: Send an email Message using SMTP and C#, we save these settings in the mailSettings section under the system.net configuration section. In this article we will make an application that can be used to load and save mail settings.
Using the Code:
To make your application send emails to customers, follow these steps:
- Create a new windows application using Visual Studio 2005/2008.
- Rename Form1 to MailSettingsForm.
- Add a Label to the MailSettingsForm and rename it to FromLabel.
- Add another Label to the MailSettingsForm and rename it to HostLabel.
- Add another Label to the MailSettingsForm and rename it to UserNameLabel.
- Add another Label to the MailSettingsForm and rename it to PasswordLabel.
- Add a TextBox to the MailSettingsForm and rename it to FromTextBox.
- Add another TextBox to the MailSettingsForm and rename it to HostTextBox.
- Add another TextBox to the MailSettingsForm and rename it to PortTextBox.
- Add another TextBox to the MailSettingsForm and rename it to UserNameTextBox.
- Add another TextBox to the MailSettingsForm and rename it to PasswordTextBox and set the PasswordChar property to *.
- Add a CheckBox to the MailSettingsForm and rename it to DefaultCredentialsCheckBox.
- Import the System.Reflection, System.Configuration and System.Net.Configuration namespaces using the following statement:
using System.Reflection;
using System.Configuration;
using System.Net.Configuration;
- The System.Reflection namespace contains types that retrieve information about assemblies, modules, members, parameters, and other entities in managed code by examining their metadata.
- The System.Configuration namespace contains the types that provide the programming model for handling configuration data.
- The System.Net.Configuration namespace contains classes that applications use to programmatically access and update configuration settings for the System.Net namespaces.
- Add the following code to the MaillsettingsForm Load event to load the mail settings at the form startup:
private Configuration config;
private MailSettingsSectionGroup mailSettings;
private int port;
private void MailSettingsForm_Load(object sender, EventArgs e)
{
try
{
config = ConfigurationManager.OpenExeConfiguration(Assembly.GetExecutingAssembly().Location);
mailSettings = (MailSettingsSectionGroup)config.GetSectionGroup("system.net/mailSettings");
FromTextBox.Text = mailSettings.Smtp.From;
HostTextBox.Text = mailSettings.Smtp.Network.Host;
PortTextBox.Text = mailSettings.Smtp.Network.Port.ToString();
UserNameTextBox.Text = mailSettings.Smtp.Network.UserName;
PasswordTextBox.Text = mailSettings.Smtp.Network.Password;
DefaultCredentialsCheckBox.Checked = mailSettings.Smtp.Network.DefaultCredentials;
}
catch (Exception ex)
{
MessageBox.Show(ex.Message, ex.GetType().ToString(),
MessageBoxButtons.OK, MessageBoxIcon.Error);
}
}
- In the above code:
- First we create an object of the Configuration class and name it config. This object represents a configuration file that is applicable to a particular computer, application, or resource.
- We then create an object of the MailSettingsSectionGroup class and name it mailSettings. This class corresponds to the mailSettings Element (Network Settings) configuration element, is used to access information about SMTP settings for the local computer and provides programmatic access to information that can be stored in configuration files.
- We then create an integer variable named port to hold the port number that we use to connect to the SMTP server.
- In the Load event of the MailSettingsForm, we initialize the config object by using the ConfigurationManager class that provides access to configuration files for client applications using the OpenExecConfiguration method and pass to it the location of the assembly that contains the code that is currently executing.
- We then initialize the mailSettings object by calling Configuration.GetSectionGroup method that is used to obtain a specific ConfigurationSectionGroup from a Configuration object and and passing to it the mailSettings section under the system.net section and casting it to MailSettingsSectionGroup object.
- Now we have the mailSettings object loaded with the settings in the configuration file.
- We set the FromTextbox text property to the mailSettings.Smtp.From property.
- We set the HostTextbox text property to the mailSettings.Smtp.Network.Host property.
- We set the PortTextbox text property to the mailSettings.Smtp.Network.Port property.
- We set the UserNameTextbox text property to the mailSettings.Smtp.Network.UserName property.
- We set the PasswordTextbox text property to the mailSettings.Smtp.Network.Password property.
- We set the DefaultCredentialsCheckBox Checked property to the mailSettings.Smtp.Network. DefaultCredentials property.
- Now you have loaded the mail settings from the configuration file and places them to the MailSettingsForm.
- Add an ErrorProvider component to the MailSettingsForm and name it errorProvider. The Error Provider provides a user interface for indication that a control on the form has an error associated with it.
- Add a button to the MailSettingsForm and name it SaveSettingsButton and double click the SaveSettingsButton in the designer to create the Click event handler in the code.
- Add the following code to the SaveSettingsButton Click Event Handler:
private void SaveSettingsButton_Click(object sender, EventArgs e)
{
try
{
errorProvider.Clear();
if (string.IsNullOrEmpty(FromTextBox.Text))
{
errorProvider.SetError(FromTextBox, "Required");
return;
}
if (string.IsNullOrEmpty(HostTextBox.Text))
{
errorProvider.SetError(HostTextBox, "Required");
return;
}
if (string.IsNullOrEmpty(PortTextBox.Text))
{
errorProvider.SetError(PortTextBox, "Required");
return;
}
else
{
if (!int.TryParse(PortTextBox.Text, out port))
{
errorProvider.SetError(PortTextBox, "Invalid");
return;
}
}
DialogResult result = MessageBox.Show("Are you sure you want to Save?",
"Are you sure you want to Save?", MessageBoxButtons.YesNo, MessageBoxIcon.Question);
if (result == DialogResult.Yes)
{
int.TryParse(PortTextBox.Text, out port);
mailSettings.Smtp.From = FromTextBox.Text;
mailSettings.Smtp.Network.Host = HostTextBox.Text;
mailSettings.Smtp.Network.Port = port;
mailSettings.Smtp.Network.UserName = UserNameTextBox.Text;
mailSettings.Smtp.Network.Password = PasswordTextBox.Text;
mailSettings.Smtp.Network.DefaultCredentials = DefaultCredentialsCheckBox.Checked;
config.Save(ConfigurationSaveMode.Modified);
MessageBox.Show("The Operation is successfully completed", "Success",
MessageBoxButtons.OK, MessageBoxIcon.Information);
}
}
catch (Exception ex)
{
MessageBox.Show(ex.Message, ex.GetType().ToString(),
MessageBoxButtons.OK, MessageBoxIcon.Error);
}
}
- In the above code:
- First of all we clear any error in the errorProvider component by calling the Clear method of the errorProvider.
- We check that the user enters a value in the FromTextBox and we notify him if no value is entered. You will see a blinking red image beside the text box if no value is entered and if you stop the mouse over it a tooltip is displayed with a required text.
- We then check that the user enters a value in the HostTextBox and we notify him if no value is entered. You will see a blinking red image beside the text box if no value is entered and if you stop the mouse over it a tooltip is displayed with a required text.
- We then check that the user enters a value in the PortTextBox and we notify him if no value is entered. You will see a blinking red image beside the text box if no value is entered and if you stop the mouse over it a tooltip is displayed with a required text. If the user types a value in the Port and this value is not an integer number we notify the user that this value is invalid.
- We then notify the user to confirm saving these settings or not.
- If the user wants to save these settings by clicking on yes, then we get the port number and set the mailSettings.Smtp.From to the text in the FromTextBox.
- Set the mailSettings.Smtp.Network.Host to the HostTextBox text property.
- Set mailSettings.Smtp.Network.Port to the port number in the PortTextBox.
- Set mailSettings.Smtp.Network.UserName to the UserNameTextbox text property.
- Set mailSettings.Smtp.Network.Password to the PasswordTextBox text property.
- We then set if these credentials are the default credentials to use when connecting to SMTP server.
- We then save the modified sections of the configuration file and tell the user that the save operation is successfully completed.
- If there is any exception, we will display a message box containing the exception message.
- Now compile and run the application.
- The mail settings will be loaded to you. Type your preferred settings and click on the Save Settings, You will be notified if the mail settings is saved or not.
Note: You can apply the techniques in the How to: Check that a server is reachable across the network using C# and How to: Telnet a remote service using C# to ping the SMTP server and test that the SMTP service is running.
Now you have an application that can load and save mail settings and this is the final look of the application:
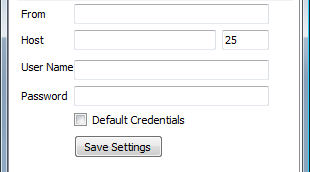
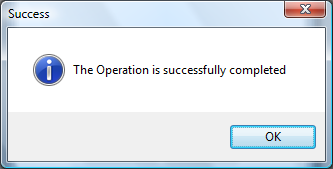